This post is in continuation with my previous post where we created an ASP.NET MVC with JQgrid. Also, as we will be using the same solution on which we worked in the last post. Versions used –
- jQuery – 1.3.2
- jqGrid – jqGrid 3.5 ALFA 3.
- jQuery UI 1.7.1
The source code of the demonstration can be downloaded here.
A jQuery Dialog box is just HTML markup tags that sit in DIV tag inside your page. This DIV tag is made invisible to the user when the user is on the page by style="display:none" attribute. This is done obviously because the user need to see this content in a dialog box only when it is invoked. Let’s start with including jQuery script files to the solution. It can be downloaded from here. I’ve added the jQuery UI scripts in a subfolder called “jQueryUI” within the Scripts folder. Now open the solution and go to Index.aspx where we have the jqGrid. On this page copy paste the following HTML tags in the MainContent place holder (it is not important where you put this tag on the page):
<div id="detailsDialog" style="display:none" title="Movie Details"> <table> <tr> <td><h2 id="movieName"></h2></td> <td> <img id="movieImage" src="" alt=""/> </td> </tr> <tr> <td>Director</td> <td id="director"></td> </tr> <tr> <td>Release Date</td> <td id="releaseDate"></td> </tr> <tr> <td>IMDB User Rating</td> <td> <a id="iMDBUserRating" target="_blank" href=""></a> </td> </tr> <tr> <td>Plot</td> <td id="plot"></td> </tr> </table> </div>
This is the set of tags that will be run by the Dialog box. Also for that we need to import the following scripts on the page:
<script src="<%= this.ResolveClientUrl("~/Scripts/jQueryUI/ui.core.js") %>" type="text/javascript"></script> <script src="<%= this.ResolveClientUrl("~/Scripts/jQueryUI/ui.draggable.js") %>" type="text/javascript"></script> <script src="<%= this.ResolveClientUrl("~/Scripts/jQueryUI/ui.resizable.js") %>" type="text/javascript"></script> <script src="<%= this.ResolveClientUrl("~/Scripts/jQueryUI/ui.dialog.js") %>" type="text/javascript"></script>Now we have the tags ready, we need some jQuery to dynamically put data in there. The jqGrid that we are using has some events associated to it like “onSortCol”, “ondblClickRow” etc. For details on these events please refer to the documentation here. The event that we will use here will be onSelectRow. As the name suggests the event will be fired when the user clicks on a row and we will create a handler for the event. Let the name of the handler be – ShowMovieDetails. The Event with the handler needs to be added to the jqGrid’s properties:
onSelectRow: ShowMovieDetailsPlease note that ShowMovieDetails is not proceeded by the parenthesis “()”. Doing so will actually execute the function. In order to assign but not execute we drop the parenthesis. This event, when fired, sends the value of the selected row number to the handler as a parameter. In our scenario the handler needs to do the following this: 1. Create a dialog box, 2. Set the values dynamically in the DIV based on the row selected. 3. Show the dialog box. To achieve this we will use a method in jqGrid to get the cell value of the grid. This method is – getCell(rowId, columnId). This can be invoked as - $(<jqGridSelector>).getCell(rowId, colId). Here’s the handler for our purpose:
function ShowMovieDetails(rowid) { $('#detailsDialog').dialog({ autoOpen: false, width: 550, height: 400, modal: true }); $("#movieName").text($("#list").getCell(rowid, 1)); $("#movieImage").attr("src", $("#list").getCell(rowid, 6)); $("#movieImage").attr("alt", $("#list").getCell(rowid, 1)); $("#director").text($("#list").getCell(rowid, 2)); $("#releaseDate").text($("#list").getCell(rowid, 3)); $("#iMDBUserRating").text($("#list").getCell(rowid, 4)); $("#iMDBUserRating").attr("href", "http://www.imdb.com/find?s=tt&q=" + $("#list").getCell(rowid, 1)) $("#plot").text($("#list").getCell(rowid, 5)); $('#detailsDialog').dialog('open'); }Please take note of the properties in the dialog. If you do not use the dialog('open') method separately to open the dialog box, the dialog box will open but only for the first time. Subsequent selection of rows will have no response. To mitigate this, we declared the dialog with autoOpen as false and open the dialog box separately using dialog('open'). Now go ahead and run the application for gratification. This is what shows up:
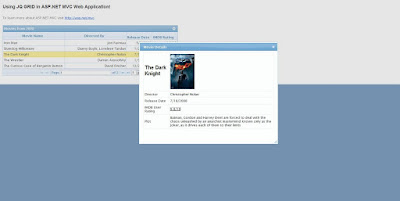
Thanks.is there anyway to display the image in the grid itself?
ReplyDeletethis is excellent example. thanks for posting the source code as well . .
ReplyDelete@firehose911 – I haven’t tried but I’m sure you will be able to do that. Try adding a column in the grid for the image. From the code behind, for the value of that column, send an image tag with values set.
ReplyDeleteI think it will work, however the image size will have to be managed.
@Anonymous – Thank you.
Se possibile grazie merluzzo fonte download di questo exaple?
ReplyDelete@Maltra - You can download the Source Code from:
ReplyDeletehttp://sites.google.com/site/aspnetlive/resources/jQueryMVC.zip